How to construct Scalable Applications for a Developer By Gustavo Woltmann
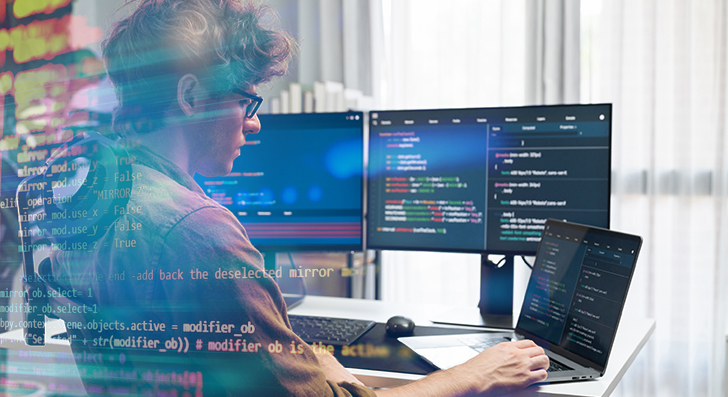
Scalability suggests your application can take care of progress—much more users, extra knowledge, and a lot more site visitors—without having breaking. As a developer, making with scalability in your mind saves time and stress afterwards. Right here’s a transparent and functional manual to help you start out by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability is not something you bolt on later on—it should be aspect of one's plan from the start. Many purposes fall short when they increase quick for the reason that the initial design can’t take care of the extra load. As a developer, you'll want to Feel early regarding how your method will behave stressed.
Commence by building your architecture for being adaptable. Keep away from monolithic codebases the place all the things is tightly connected. As a substitute, use modular style or microservices. These designs crack your app into more compact, independent sections. Every module or provider can scale By itself without impacting The full procedure.
Also, consider your database from day one. Will it want to manage one million buyers or simply a hundred? Select the right kind—relational or NoSQL—depending on how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
An additional vital stage is to prevent hardcoding assumptions. Don’t generate code that only operates beneath present-day conditions. Take into consideration what would come about If the person foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that assistance scaling, like message queues or occasion-driven systems. These help your application deal with much more requests with out obtaining overloaded.
Whenever you Develop with scalability in mind, you are not just planning for achievement—you are cutting down long run complications. A effectively-planned procedure is less complicated to keep up, adapt, and expand. It’s much better to prepare early than to rebuild later.
Use the Right Databases
Picking out the proper database is often a critical Section of developing scalable purposes. Not all databases are designed a similar, and utilizing the Improper you can sluggish you down or perhaps induce failures as your application grows.
Commence by comprehending your details. Could it be very structured, like rows in a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient match. These are definitely sturdy with relationships, transactions, and regularity. Additionally they support scaling approaches like go through replicas, indexing, and partitioning to handle additional site visitors and details.
Should your data is much more adaptable—like consumer activity logs, merchandise catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at handling huge volumes of unstructured or semi-structured details and may scale horizontally extra very easily.
Also, think about your read through and write patterns. Will you be doing a great deal of reads with much less writes? Use caching and read replicas. Have you been dealing with a major produce load? Consider databases that will cope with high compose throughput, or maybe party-based info storage programs like Apache Kafka (for non permanent info streams).
It’s also sensible to Imagine in advance. You may not require Innovative scaling capabilities now, but deciding on a databases that supports them indicates you won’t want to modify afterwards.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your information dependant upon your entry designs. And generally watch databases general performance as you expand.
In a nutshell, the best database is dependent upon your app’s construction, speed wants, And the way you anticipate it to develop. Acquire time to choose properly—it’ll preserve plenty of problems later.
Optimize Code and Queries
Speedy code is essential to scalability. As your application grows, every single modest hold off provides up. Badly composed code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s important to Establish economical logic from the beginning.
Commence by writing clean, straightforward code. Avoid repeating logic and take away everything needless. Don’t choose the most elaborate Option if a simple one works. Maintain your functions brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—locations where by your code normally takes also long to operate or makes use of too much memory.
Future, have a look at your database queries. These typically slow points down greater than the code alone. Make certain Each individual query only asks for the info you actually will need. Prevent Choose *, which fetches all the things, and as an alternative find distinct fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, Specially throughout big tables.
When you notice a similar info staying requested repeatedly, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your databases functions after you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and would make your application much more productive.
Remember to take a look at with significant datasets. Code and queries that work fantastic with one hundred records may crash after they have to manage one million.
Briefly, scalable applications are speedy applications. Keep the code limited, your queries lean, and use caching when needed. These steps aid your application keep clean and responsive, at the same time as the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to manage additional buyers plus more traffic. If every thing goes via a single server, it's going to promptly turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources assist keep your application speedy, secure, and scalable.
Load balancing spreads incoming website traffic throughout a number of servers. As opposed to a single server executing every one of the operate, the load balancer routes consumers to various servers according to availability. This suggests no one server will get overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this straightforward to set up.
Caching is about storing details briefly so it can be reused immediately. When people request exactly the same information all over again—like an item web page or simply a profile—you don’t ought to fetch it in the databases when. It is possible to serve it with the cache.
There are two popular varieties of caching:
one. Server-aspect caching (like Redis or Memcached) suppliers knowledge in memory for quick entry.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near the consumer.
Caching cuts down database load, increases speed, and can make your app far more economical.
Use caching for things that don’t improve usually. And generally make certain your cache is up-to-date when data does improve.
In a nutshell, load balancing and caching are very simple but potent instruments. Together, they help your application handle a lot more people, stay quickly, and Get well from problems. If you plan to increase, you would like both equally.
Use Cloud and Container Tools
To construct scalable apps, you would like tools that let your app increase conveniently. That’s where cloud platforms and containers are available in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to obtain components or guess long run potential. When targeted visitors increases, you are able to add much more sources with only a few clicks or instantly making use of vehicle-scaling. When targeted visitors drops, you can scale down to economize.
These platforms also give products and Gustavo Woltmann blog services like managed databases, storage, load balancing, and stability applications. You could deal with making your application as an alternative to controlling infrastructure.
Containers are Yet another important Instrument. A container deals your application and anything it should run—code, libraries, settings—into a person device. This causes it to be straightforward to move your application amongst environments, out of your laptop into the cloud, devoid of surprises. Docker is the most well-liked Instrument for this.
Once your application utilizes a number of containers, resources like Kubernetes help you regulate them. Kubernetes handles deployment, scaling, and Restoration. If 1 section of your respective app crashes, it restarts it quickly.
Containers also ensure it is easy to different areas of your application into expert services. You'll be able to update or scale pieces independently, that's great for general performance and trustworthiness.
In brief, applying cloud and container equipment means it is possible to scale fast, deploy quickly, and recover promptly when issues transpire. If you'd like your application to develop with no limits, start off using these equipment early. They help you save time, decrease possibility, and allow you to continue to be focused on constructing, not correcting.
Keep track of Almost everything
If you don’t check your software, you received’t know when things go Improper. Checking allows you see how your app is doing, location issues early, and make far better selections as your application grows. It’s a vital Section of setting up scalable systems.
Commence by monitoring primary metrics like CPU use, memory, disk space, and response time. These let you know how your servers and providers are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this info.
Don’t just check your servers—keep an eye on your application far too. Regulate how much time it's going to take for buyers to load internet pages, how often mistakes take place, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for significant challenges. One example is, If the reaction time goes previously mentioned a limit or perhaps a services goes down, you need to get notified instantly. This assists you fix issues speedy, normally before users even see.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again prior to it results in authentic injury.
As your app grows, targeted visitors and knowledge improve. Without checking, you’ll skip indications of difficulties right up until it’s as well late. But with the ideal equipment in place, you keep in control.
To put it briefly, monitoring allows you maintain your application reputable and scalable. It’s not just about spotting failures—it’s about understanding your technique and making sure it really works well, even stressed.
Final Feelings
Scalability isn’t only for huge providers. Even modest applications want a solid foundation. By building meticulously, optimizing sensibly, and using the suitable tools, it is possible to build apps that mature smoothly with no breaking stressed. Commence smaller, think huge, and Make intelligent.